Guide to For Loops in Python and Bash [Explained with Examples]
What is a For Loop?
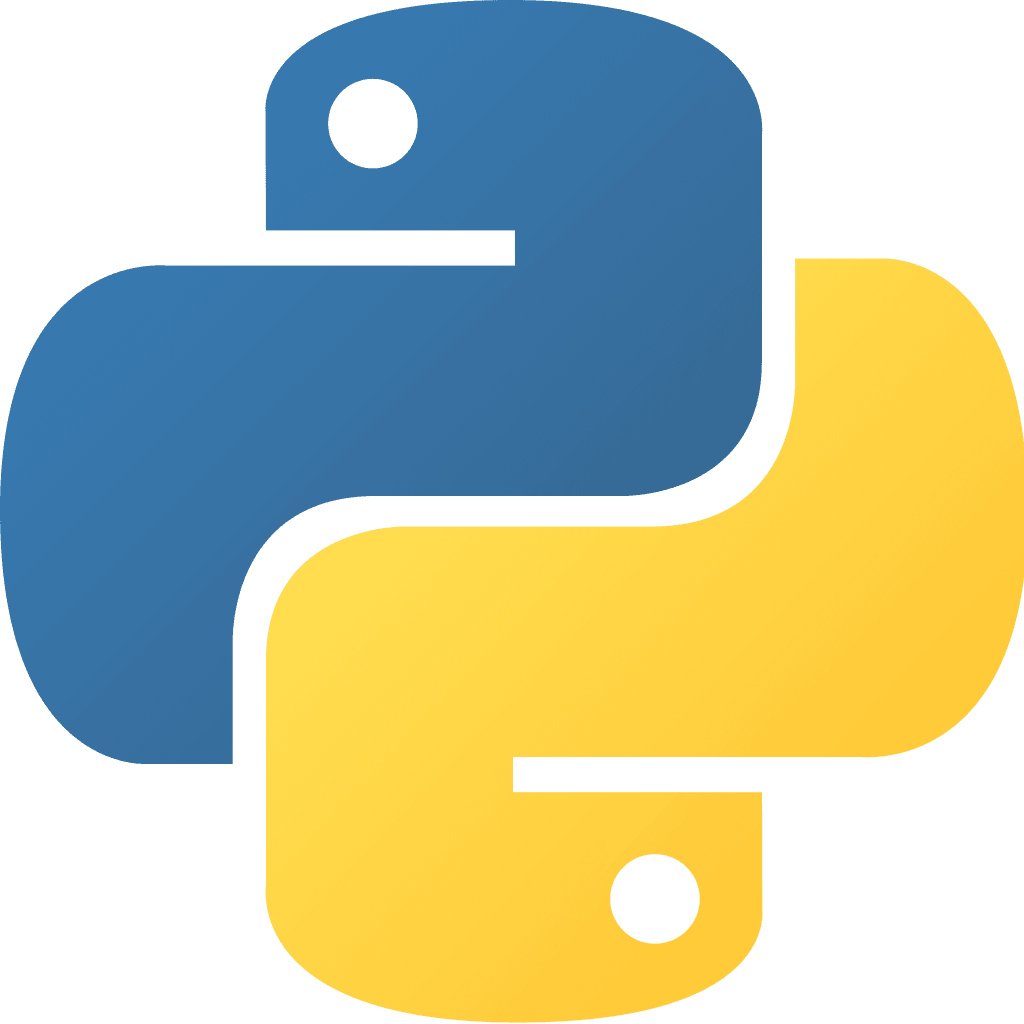
A for loop in Python is utilized to make repeated use of a function or procedure, applying it each time to the result of the previous sequence. This repeating process is called iteration. The for loop checks each iteration until a condition is met. Typically these are used when we have to run a block of code duplicating it X number of times. Each time it iterates over a sequence, it re-executes the code.
Syntax of a For Loop
The typical syntax of a for loop in a python script is seen below.
#!/usr/bin/python3
for a in sequence:
body of for
How Does a For Loop Work
Multiple languages have for loop syntax options within their code. In Python, any object with a method that is iterable can be utilized in a for loop. An iterable method is defined as data presented in the form of a list, where multiple values exist in an orderly manner. We can also define our iterables arrays by creating objects using the next() or iter() methods.
Code Examples
Using a Simple For Loop
A Pythonfor statement runs a sequence of code over and over again, in order, executing the same code block each time. This function allows us to re-run a command X number of times until we reach the desired result. The alternative looping command uses the while statement. Both of these constructs are used for different purposes. Here, we use (0,3) to determine the number of iterations to run.
#!/usr/bin/python3
#simple for loop example
for x in range(0, 3):
print("Loop %d" % (x))
The command’s output is below.
root@host:~# python forloop1.py
Loop 0
Loop 1
Loop 2
root@host:~#
We can see that the for loop runs for a fixed number of iterations (0, 3) while adding a number for each loop completed. In the next example, the while statement (using the boolean value True) never changes.
Using a Range Statement in a For Loop
The range() function has two main sets of parameters:
- range(stop)
- Stop indicates the quantity of whole numbers (or integers) to render, starting from 0.
- range([start], stop[, step])
- start: This is the beginning number of the sequence.
- stop: Numbers are generated up to, but not including, this number.
- step: The difference between the numbers within the sequence.
Employing the range() function in a script, we can define a set number of loops to iterate through that sequence. As an example, we can define the range as range(12). Because Python defines 0 as the first number in a range (or list of indexes), our example range is 0 to 11, not 1 to 12. Python is 0-index based. This means that a list of indexes begins at 0, not 1. Lastly, users should note that all parameters should be positive or negative integers.
As an example, specifying the start and stop points in a range() function like the one below, a list will be generated from 4 to 7 (5 numbers).
range(4,7)
Using the forloop4.py script, we can generate a two-parameter range.
#!/usr/bin/python3
for i in range(4, 7):
print(i)
The script output is below.
root@host:~# python forloop4.py
4
5
6
root@host:~#
Using the following forloop3.py script, we can output a single list of numbers 0-6.
#!/usr/bin/python3
for i in range(7):
print(i)
The script output is below.
root@host:~# python forloop3.py
0
1
2
3
4
5
6
root@host:~#
Using this same method, we can use the forloop5.py script to increment a list of negative numbers, going by threes.
#!/usr/bin/python3
for i in range(0, -12, -3):
print(i)
The script output is below.
root@host:~# python forloop5.py
0
-3
-6
-9
root@host:~#
Using a For Loop Statement on a String
Strings can produce a succession of characters because they are considered iterable objects. Using the forloop6.py script, we can list the string in a sequence.
#!/usr/bin/python3
for x in "watermelon":
print(x)
The script output is below.
root@host:~# python forloop6.py
w
a
t
e
r
m
e
l
o
n
root@host:~#
Using a Break Statement in a For Loop
When using a break statement, we can stop a loop before it has a chance to loop through all our listed items. Using the forloop7.py script, we can terminate the script when the loop reaches the Chevy entry.
#!/usr/bin/python3
cars = ["Ford", "Chevy", "GM"]
for x in cars:
print(x)
if x == "Chevy":
break
The script output is below.
root@host:~# python forloop7.py
Ford
Chevy
root@host:~#
Using a Continue Statement in a For Loop
If we use the continue statement, we can cease the current iteration loop and continue with the following entry. Using the forloop8.py script that employs our previous cars example will stop the current iteration loop and continue with the next entry in the list.
#!/usr/bin/python3
cars = ["Ford", "Chevy", "GM"]
for x in cars:
if x == "Chevy":
continue
print(x)
Output
root@host:~# python forloop8.py
Ford
GM
root@host:~#
Using an Else Statement in a For Loop
The else statement in a for loop runs a specific block of code when the loop completes. Using the forloop9.py script, we can output a range and then print the message “Done” when it finishes. Users should note that the else block will not be executed if a break statement halts the running loop.
#!/usr/bin/python3
for x in range(5):
print(x)
else:
print("Done.")
Output.
root@host:~# python forloop9.py
0
1
2
3
4
Done.
root@host:~#
Using a Nested Loop Inside a For Loop
A nested loop is simply a loop within a loop. The inside loop will run once for each iteration of the outer loop. Using the forloop10.py script, we
#!/usr/bin/python3
adj = ["Blue", "Yellow", "Red"]
cars = ["Ford", "Chevy", "GM"]
for x in adj:
for y in cars:
print(x, y)
Output.
root@host:~# python forloop10.py
('Blue', 'Ford')
('Blue', 'Chevy')
('Blue', 'GM')
('Yellow', 'Ford')
('Yellow', 'Chevy')
('Yellow', 'GM')
('Red', 'Ford')
('Red', 'Chevy')
('Red', 'GM')
root@host:~#
Using a Pass Statement in a For Loop
Technically, for loops should not be left empty. If we must leave a for loop empty, we can use a pass statement to prevent errors. Using the forloop11.py script, we should get no output at all when running our script.
#!/usr/bin/python3
for x in[1, 2, 3]:
pass
Output.
root@merovingian2:~# python forloop11.py
root@merovingian2:~#
If we remove the pass statement, here is the output.
root@host:~# python forloop11.py
File "forloop11.py", line 3
^
IndentationError: expected an indented block
root@host:~#
Different Iterable Data Types with Python For Loop Examples
For Loop over List collection
List is a built-in data type in Python whose Items are ordered, changeable, and can have duplicate values. List values are indexed from 0, and when new items are added on the end of a list. Here is an example of how to iterate through List using For Loop where we added one more value using append method.
#!/usr/bin/env python3
animal = ["cow","dog","cat","elephant"]
animal.append("bird")
for name in animal:
print (name)
Output.
cow
dog
cat
elephant
bird
For Loop over Tuple collection
Tuple is a built-in iterable data type that is ordered,unchangeable, and can have duplicate values. Tuple values are indexed from 0 same as List, the only difference is that we can’t add values after we create a Tuple. Tuples are iterated the same way as Lists are.
#!/usr/bin/env python3
animal = ("cow","dog","cat","elephant")
#animal.append("bird")
for name in animal:
print (name)
Output.
cow
dog
cat
elephant
And if we uncomment append function on line 3, we will get an error.
Traceback (most recent call last):
File "main.py", line 3, in <module>
animal.append("bird")
AttributeError: 'tuple' object has no attribute 'append'
For Loop over a Set collection
Set is a built-in iterable data type that is unordered, unchangeable, and can not have duplicate values. While specific items can’t be changed in a set, you can still remove and add items to it. Since Sets are unordered when we use it in a for loop we are going to get different results each time we run the script.
#!/usr/bin/env python3
animal = {"cow","dog","cat","elephant"}
animal.add("bird")
for name in animal:
print (name)
Output on the first run.
cow
dog
cat
bird
elephant
Output on the second run.
bird
cow
elephant
dog
cat
For Loop over a Frozenset collection
Frozenset is a built-in Python function that converts iterable objects such as list or tuple, and makes it into a set but unchangeable. Once we use the Frozenset function on an iterable object we won’t be able to add, remove or change an item.
#!/usr/bin/env python3
animal = ["cow","dog","cat","elephant"]
animal.append("bird")
#bird has been added to animal list
frset = frozenset(animal)
for name in frset:
print (name)
Output on the first run.
cat
bird
cow
elephant
dog
Output on the second run.
dog
elephant
bird
cat
cow
For loop over a Dictionary collection
Dictionary is a built-in data type in Python that is ordered, changeable, and can not have duplicate values. Dictionary stores items in key-value pairs, and values can be accessed by using key names. Using a For Loop we can iterate through keys, values, or both.
#!/usr/bin/env python3
animal = {"type":"cat","name":"Felix","Age":"3"}
#Iterating through keys
for x in animal.keys():
print (x)
#Iterating through values
for x in animal.values():
print (x)
#Iterating through both
for x,y in animal.items():
print (x,y)
Output.
type
name
Age
cat
Felix
3
type cat
name Felix
Age 3
For Loop over Enumerate
Enumerate is a built-in function that allows you to input an iterable type which then returns the count number and value into a tuple that has key value data type. Let's see an example of enumerating a List using a For Loop.
#!/usr/bin/env python3
animal = ["cow","dog","cat","elephant"]
for tup in enumerate(animal):
print (tup)
Output.
(0, 'cow')
(1, 'dog')
(2, 'cat')
(3, 'elephant')
Every iteration of this script is going to be the same because we used a List type as input. Let's see what happens when we input Set type into the enumeration.
#!/usr/bin/env python3
animal = {"cow","dog","cat","elephant"}
for tup in enumerate(animal):
print (tup)
Output on the first run.
(0, 'cat')
(1, 'cow')
(2, 'dog')
(3, 'elephant')
Output on the second run.
(0, 'dog')
(1, 'cat')
(2, 'elephant')
(3, 'cow')
We can see that if we input Set type in enumerate, every time we run the script, the order is going to be different because of the Set being an unordered collection.
For Loop over a Zip
Zip is a built-in function that is used for parallel iteration. It combines one or more iterable data types and returns a tuple whose n-th item is the n-th item of each collection inputted in zip.
#!/usr/bin/env python3
animal = ["cow","dog","cat","elephant"]
length = range(len(animal)) #0,1,2,3
for tup in zip(length,animal):
print (tup)
Output.
(0, 'cow')
(1, 'dog')
(2, 'cat')
(3, 'elephant')
Let's see what happens if we input collections of different lengths.
#!/usr/bin/env python3
animal = ["cow","dog","cat","elephant"] #4 length
length = range(6) #7 length
fruit = ("Apple","Banana","Avocado","Mango","Cherry") #5 length
for tup in zip(length,animal,fruit):
print (tup)
Output.
(0, 'cow', 'Apple')
(1, 'dog', 'Banana')
(2, 'cat', 'Avocado')
(3, 'elephant', 'Mango')
We can see that zip is going to return a tuple with the size of the smallest collection.
10 Real-World Uses of For Loops in Bash
The examples below (or some iteration of) are often used in everyday Linux administration. Some of them may be old or reference functions that are no longer available, but they are listed to show the versatility of using a for loop in a script or terminal. Below, we rename all the files in a folder to file1, file 2, etc...
j=1; for i in *; do mv "$i" File$j; j=$((j+1)); done
Change the ownership for everything under the directory agora/ for all users in the public_html/agora folder
for i in `ls -1 /var/cpanel/users/`; do chown -R $i. /home*/$i/public_html/agora/*; done
Sometimes semaphores get stuck when Apache fails to clean up after itself. Below, we run the ipcs -s command to check on this. Clear them out with this command.
for i in `ipcs -s | awk '/httpd/ {print $2}'`; do (ipcrm -s $i); done
for i in $(ls -A .); do rm -f $i; done -
This is how to check for a particular account backup in daily/weekly/monthly folders if present, all at the same time.
for i in $(find /backup/cpbackup/ -type d | grep -i [A-Z]);do ll $i| grep -i <keyword>; done
Below shows how we can to optimize all tables in a database.
for i in $(mysql -e "show databases;" | sed 's/Database//') ; do for each in $(mysql -e "use $i; show tables;" \
| sed 's/Tables.*//' ;) ; do mysql -e "use $i ; optimize table $each" ; done ; done
Before cPanel had a visual SPF installer in WHM, we used this command to add SPF records.
for i in `ls /var/cpanel/users` ;do /usr/local/cpanel/bin/spf_installer $i ;done
Or, if we wanted to install both SPF and DKIM records, we used this command.
for i in `ls /var/cpanel/users|grep -v "./"`; do /usr/local/cpanel/bin/dkim_keys_install && /usr/local/cpanel/bin/spf_installer $i ; done ;
Here, we use a for loop to search the exim mainlog for a sender who was using three specific domains via their IP, and once found, block them in the firewall while adding a comment.
for i in $(grep -i sendername /var/log/exim_mainlog |egrep 'domain.com| domain.net | domain.org | grep "<="| egrep "([0-9]{1,3}\.){3}[0-9]{1,3}" -o | grep -v 72.52.178.16 | sort |uniq); do csf -d $i per ticket 4224475 for sending spam ;done
Here we use a for loop to kill -9 nobody PHP processes and then restart apache.
for i in $(ps aux |grep nobody |awk '{print $2}');do kill -9 $i;done && service httpd restart
Conclusion
As we can see, for loops are used in multiple situations directly from the commandline or in a script. To recap, a for loop is a flow control statement used to continuously iterate a specific code block repeatedly for a fixed number of times. We define this Python statement which frequently performs a set of conditions until acted upon by an external state or influence. Overall, Python for loops offers some of the most useful options in a modern programming language.
Liquid Web offers VPS Hosting, Cloud Dedicated Server, or Dedicated Server options for your next Python project. There are different management and configuration options to fit your needs. Contact the sales team for additional information.
Related Articles:

About the Author: Mike James
Mike is a Managed Applications Support Technician. He is a highly curious and self-motivated technology, psychology, and medicine enthusiast passionate about automating, organizing, and optimizing everyday processes. In his spare time, he enjoys gaming.
Our Sales and Support teams are available 24 hours by phone or e-mail to assist.
Latest Articles
How does Apache Web Server work? An FAQ
Read ArticleEmail security best practices for using SPF, DKIM, and DMARC
Read ArticleLinux dos2unix command syntax — removing hidden Windows characters from files
Read ArticleHow to use kill commands in Linux
Read ArticleChange cPanel password from WebHost Manager (WHM)
Read Article